728x90
반응형
조건문과 반복문의 종류를 괄호 ( ) 속에 넣어보세요.
° 조건문 : ( if ), ( switch )
° 반복문 : ( for ), ( while ), ( do-while )
조건문과 반복문을 설명한 것 중 틀린 것은 무엇입니까?
1. if문은 조건식의 결과에 따라 실행 흐름을 달리할 수있다.
2. switch문에서 사용할 수 있는 변수의 타입은 int, double이 될 수 있다.
3. for문은 카운터 변수로 지정한 횟수만큼 반복시킬 때 사용할 수 있다.
4. break문은 switch문, for문, while문을 종료할 때 사용할 수 있다.
switch문은 double형 변수를 사용할 수 없다. byte, char, short, int, long, String 타입만 가능
for문을 이용해서 1부터 100까지의 정수 중에서 3의 배수의 총합을 구하는 코드를 작성해보세요.
public class Main {
public static void main(String[] args) {
int sum = 0;
for(int i=1; i<=100; i++) {
if(i % 3 == 0) sum += i;
}
System.out.println(sum);
}
}
Math.random()메소드를 이용하여 주사위 눈의 합이 5가 되면 멈추는 코드를 작성해보세요.
public class Main {
public static void main(String[] args) {
int dice1 = 0, dice2 = 0;
while (dice1 + dice2 != 5) {
dice1 = (int)(Math.random() * 6) + 1;
dice2 = (int)(Math.random() * 6) + 1;
System.out.println("주사위1: " + dice1 + " 주사위 2: " + dice2 + " 합: " + (dice1 + dice2));
}
}
}
중첩 for문을 이용하여 방정식 4x + 5y = 60의 모든 해를 구해서 (x, y) 형태로 출력해보세요. 단, x와 y는 10이하의 자연수입니다.
public class Main {
public static void main(String[] args) {
for(int x = 1; x <= 10; x++) {
for(int y = 1; y <= 10; y++) {
if(4 * x + 5 * y == 60) System.out.printf("(%d, %d)\n", x, y);
}
}
}
}
for문을 이용해서 실행 결과와 같은 삼각형을 출력하는 코드를 작성해보세요.
public class Main {
public static void main(String[] args) {
for(int i=1; i<=5; i++) {
System.out.println("*".repeat(i));
}
}
}
while문과 Scanner를 이용해서 키보드로부터 입력된 데이터로 예금, 출금, 조회, 종료 기능을 제공하는 코드를 작성해보세요.
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
boolean run = true;
int balance = 0;
int select = 0;
Scanner scanner = new Scanner(System.in);
while(run) {
System.out.println("------------------");
System.out.println("1. 예금 | 2. 출금 | 3. 잔고 | 4. 종료");
System.out.println("------------------");
System.out.print("선택> ");
select = scanner.nextInt();
if(select == 1) {
System.out.print("예금액> ");
balance += scanner.nextInt();
} else if(select == 2) {
System.out.print("출금액> ");
balance -= scanner.nextInt();
} else if(select == 3) {
System.out.println("잔고> " + balance);
} else {
run = false;
}
}
System.out.println("프로그램 종료");
}
}
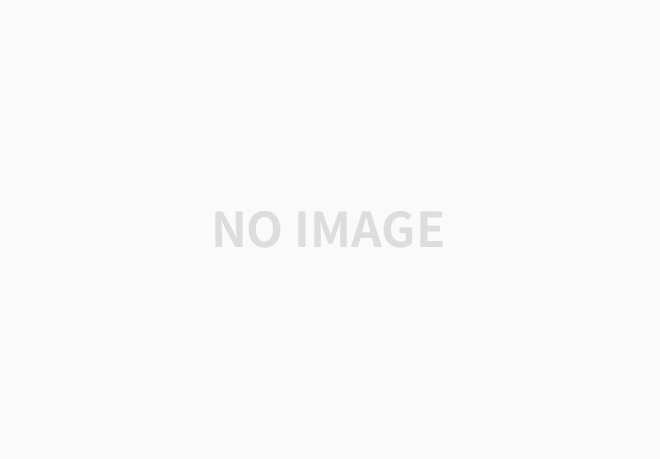
728x90
반응형
'프로그래밍 > Java' 카테고리의 다른 글
이것이 자바다 8장 확인 문제 답 - 신용권의 Java 프로그래밍 정복 (0) | 2022.10.18 |
---|---|
이것이 자바다 7장 확인 문제 답 - 신용권의 Java 프로그래밍 정복 (0) | 2022.10.18 |
이것이 자바다 6장 확인 문제 답 - 신용권의 Java 프로그래밍 정복 (0) | 2022.10.13 |
이것이 자바다 5장 확인 문제 답 - 신용권의 Java 프로그래밍 정복 (1) | 2022.10.05 |
이것이 자바다 3장 확인문제(연습문제) 답 - 신용권의 Java 프로그래밍 정복 (0) | 2022.09.27 |